Web Notification API
examples
Author @BartoszBobin
What is Web Notification?
How to use it?
with Notification object
new Notification('Notification Title', options);
* Doesn't work on mobile Chrome
with Webworkers
navigator.serviceWorker.register('sw.js', options);
navigator.serviceWorker.ready.then((registration) => {
registration.showNotification('Notification Title');
});
* Doesn't work on Edge
** Works only over localhost or HTTPS
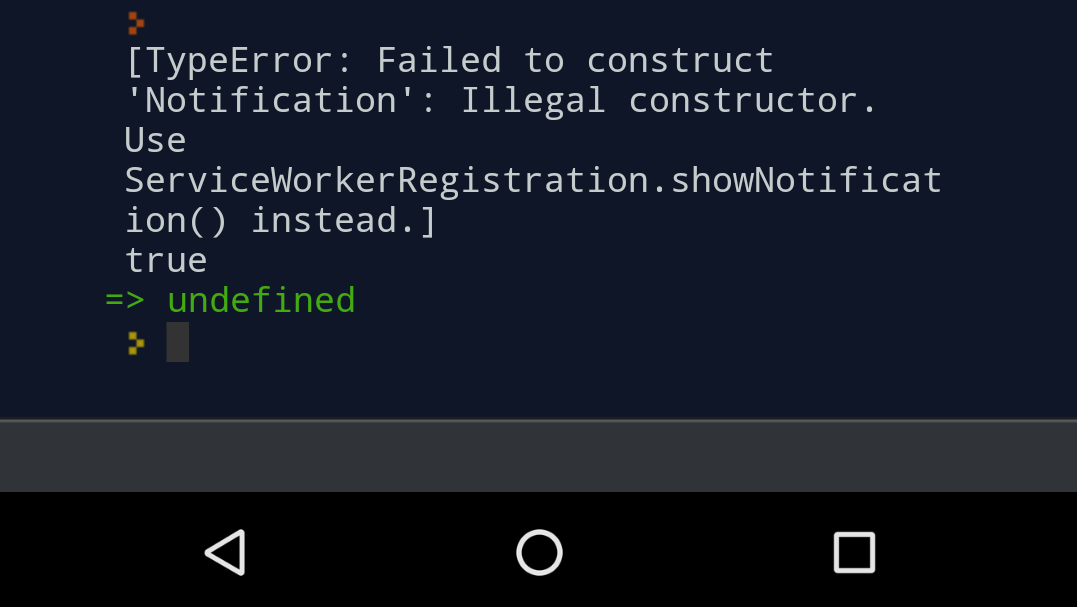
Notification API
new Notification('Notification Title', {
body: 'This notification have additional text and icon',
icon: 'smile.png',
data: {},
requireInteraction: true,
vibrate: [400, 300, 300, 800],
tag: 'group-A',
sound: 'sound.mp3'
});
Do I have permissions?
We can easy check if user allow notification for our website by checking property:
Notification.permission;
Possible values:
- default - there is no decision yet
- granted - user allow notification
- denied - user don't want to see our notification
Ask me about permissions
Before we show any notification to our user we need to ask him about permission:
Notification.requestPermission().then((result) => { });
!important:
Web browser will ask user about permission to show notification. When user will take decision eg.: denied, web browser will not ask him again.
Say Hello!
Let's try simple notification
new Notification('Hi desktop user!');
More complex example!
Let's add body text and icon to our notification...
new Notification('Extended notification', {
body: 'This notification have additional text and icon',
icon: 'smile.png'
});
More about icons
We can use PNG, JPG and GIF as an images.
What about BASE 64?
What about SVG?
How to pass extra data with Notification?
new Notification('Notification with extra object data', {
body: 'This notification have extra data',
data: {
some: 'extra-data',
2: true,
array: ["x", true, 1]
}
});
ok ... but how to deal with this extra data?
const notification = new Notification(...);
console.log(notification.data);
and inside webworkers
self.onnotificationclick = (event) => {
console.log(event.notification.data);
};
How it behave when webbrowser is not on top?
setTimeout(() => {
new Notification('Delayed notification', options);
}, 5000);
Please minimalize your browser or make another program active.
Notification will appear after 5 secs
Require interaction (desktop)
Sometimes we really want to notify user about something important and we don't want to hide our notification without user interaction ...
new Notification('Desktop - require interaction', {
requireInteraction: true
);
Notification click event
const notification = new Notification('Notification events');
notification.onclick = function (event) {
// do something ...
};
and inside webworkers
self.onnotificationclick = (event) => {
console.log(event.notification);
};
Notification with vibration and custom sound
We also can use vibration and custom sound for our notification
new Notification('Notification with vibration and custom sound', {
vibrate: [400, 300, 300, 800],
sound: 'sound.mp3',
silent: false
});
[400, 300, 300, 800] - means: 400ms vibration, 300ms pause, 300ms vibration and 800ms pause
Many notifications - too many ...
Let's imagine that user received 12 messages and every time we want to notify him ...
You will get 12 notifications with 1s delay
Group notifications by tagging
We can easy replace previous notification by giving them tag
new Notification('Tagged Notification', {
tag: 'private-message',
renotify: true
});
You will get 20 notifications with 1s delay.
Custom actions
We can also allow user to do something more than only clicking on whole notification, we can introduce buttons/actions with icon and do appropriate action
registration.showNotification('Notification with actions', {
actions: [
{action: 'action-1', title: '1st action', icon: 'smile-blue.png'},
{action: 'action-2', title: '2nd action', icon: 'smile-red.png'}
]
});
How to detect support?
We should prefer Service Workers over Notification instance - they seems to be more powerful
if ('serviceWorker' in navigator && 'Notification' in window) {
// use serviceWorkers
} else if ('Notification' in window) {
// use new Notification()
} else {
// no support for Notification
}
Browser support
Notification API is available in
Desktop | Mobile | |
---|---|---|
Chrome | ✓ | ✓ |
Firefox | ✓ | ✓ |
Edge | ✓ | - |
Safari | ✓ | - |
IE11 | × | × |
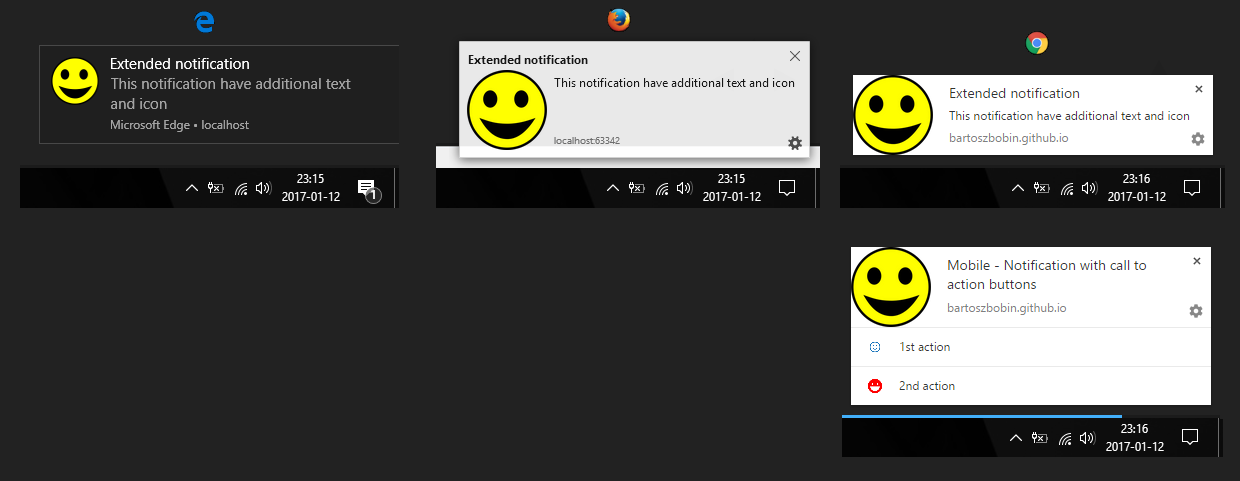
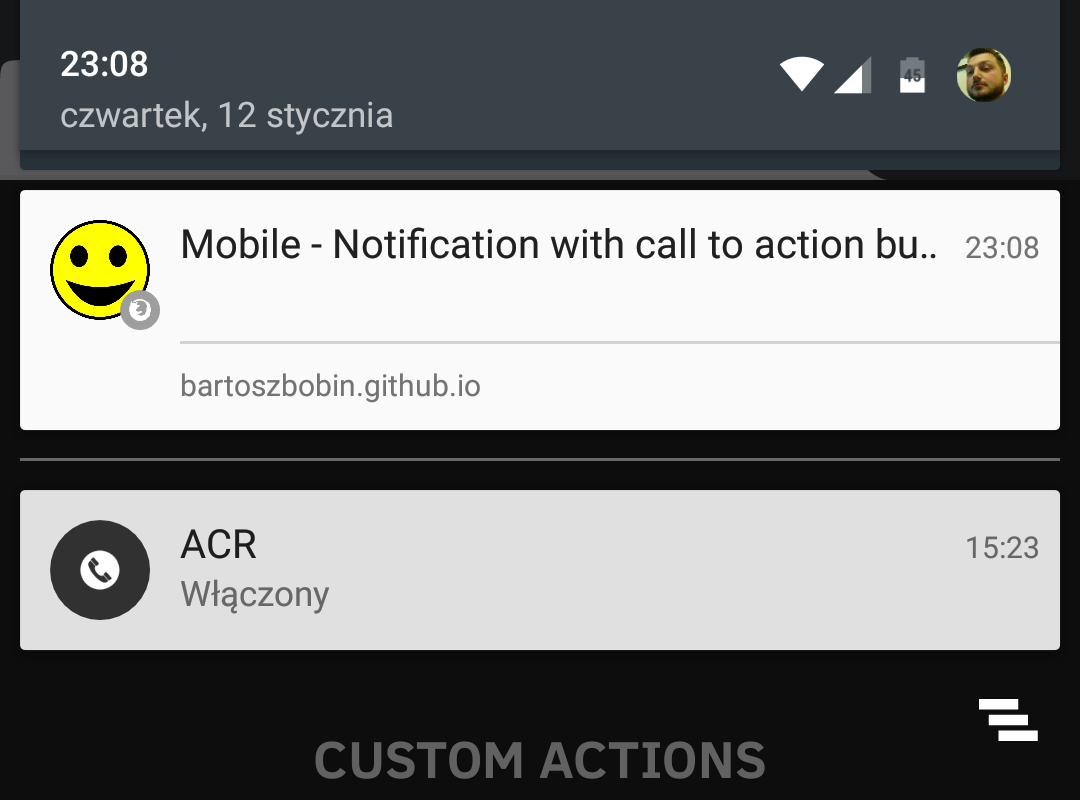
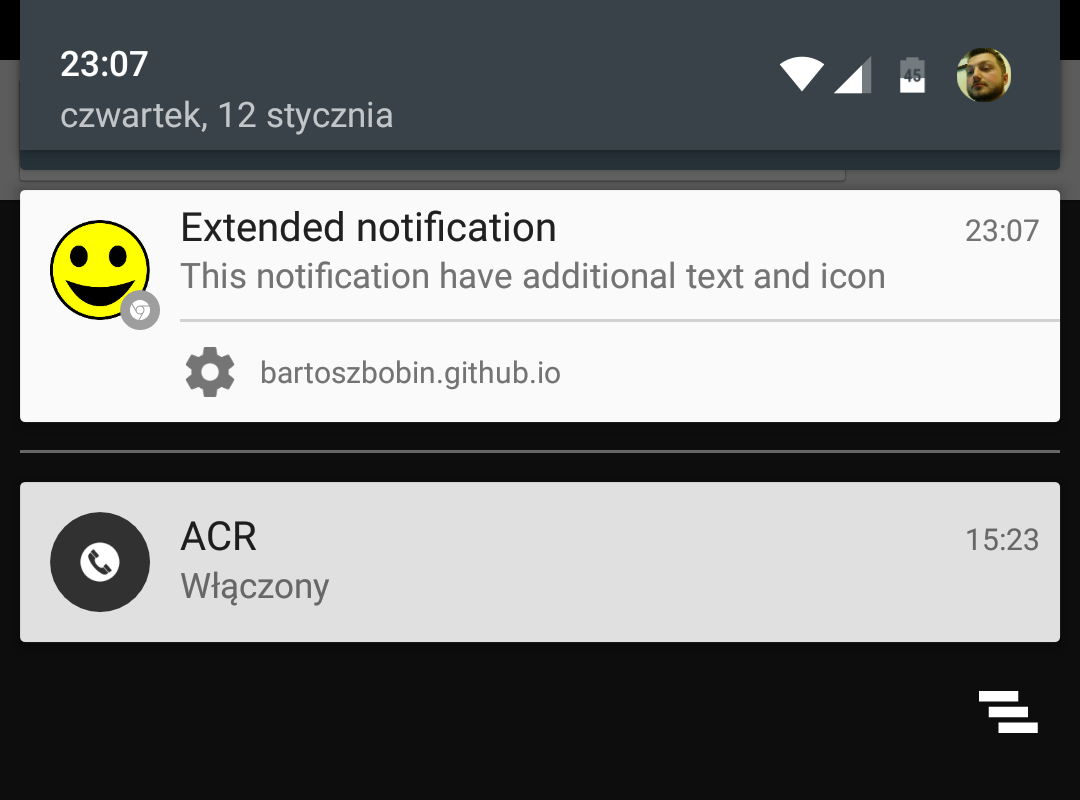
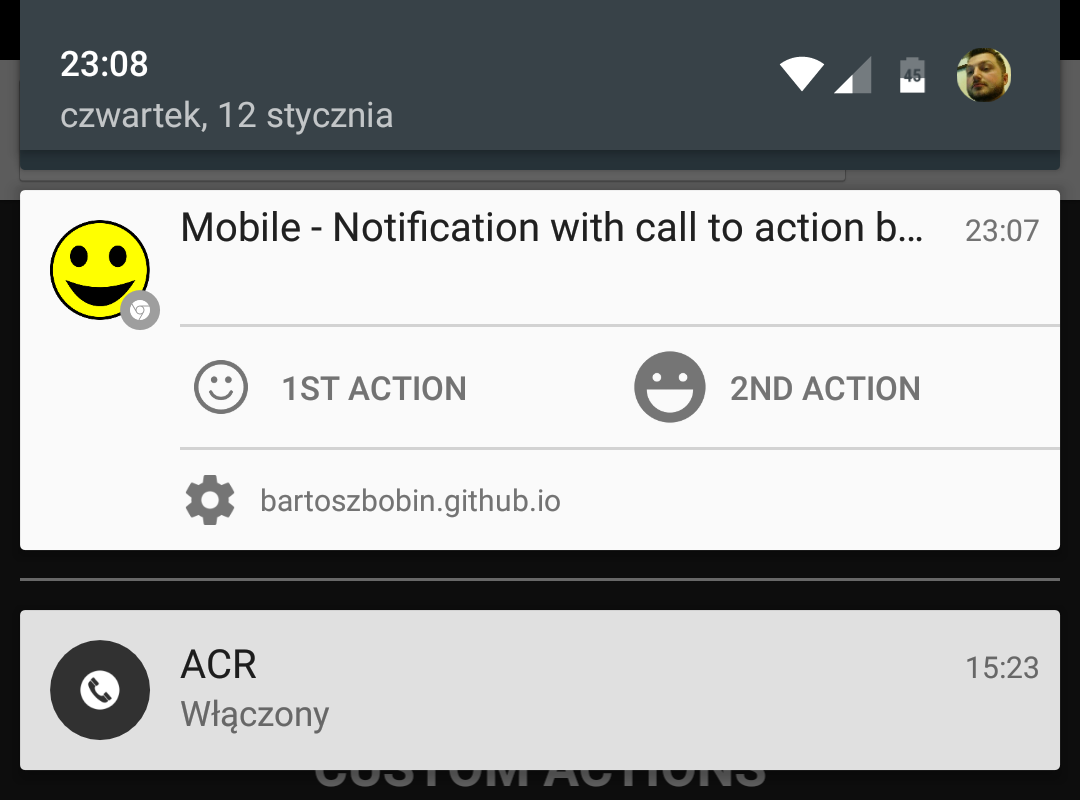